Abstract: In this piece 100x Group grantee Jeremy Rubin describes a smart contract that can be used to verifiably commit to losing coins if Taproot does not activate by a certain date. Jeremy explains a novel and interesting methodology of using smart contracts on Bitcoin, in order to conduct these “bets”. However, in our view, in practise this limited type of betting is unlikely to make a significant contribution to resolving Bitcoin’s apparent softfork activation methodology predicament.
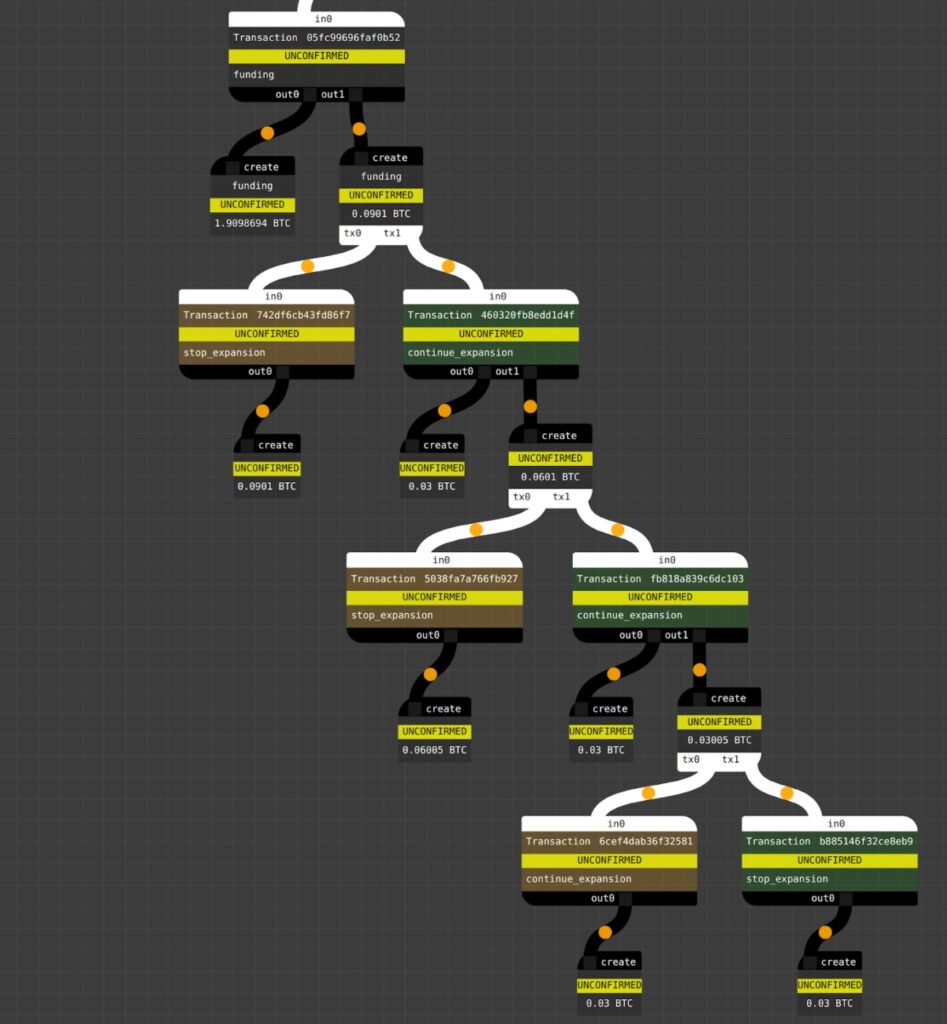
Smart Contracts for Signaling Intent to Activate Taproot
Unless you’ve been living under a rock, you’ve heard that Taproot is a currently proposed soft-fork upgrade nearing release in Bitcoin. There is nearly universal agreement that Taproot is an excellent addition to Bitcoin, however, there has been wide disagreement over how to coordinate activating the upgrade.1
For those of you familiar with my previous explorations of Probabilistic Soft Forks, you’ll know that I’m a fan of activation mechanisms that require skin in the game. In this post, I’ll describe how in a User Activated Soft Fork, Bitcoin holders can verifiably commit to losing coins2 if Taproot does not activate, which honestly signals a commitment to only recognize a chain which enforces Taproot.
This post serves a dual-purpose; I mainly wanted to show off Sapio, the Bitcoin Smart Contract Programming Language I’ve been developing. Sapio is designed to work with BIP-119 CheckTemplateVerify (CTV), a proposed extension to Bitcoin, but this contract in particular does not require CTV to work as it is able to use self-presigned transactions without majorly affecting the bindingness of the contract. I’ll be releasing Sapio for public use soon, but if you want early access, DM me on Twitter (@JeremyRubin).
The idea for a user-generated costly signal came from a conversation with Madars Virza, one of the founding scientists behind Zcash. In particular, Madars asked me if I could design a contract which allows someone to automatically place a series of bets — say, aligned with 2,016 block BIP8/BIP9 signaling periods — on whether or not Taproot was active (by spreading the bet over a longer window, you can hedge the total risk if you aren’t sure the exact period it would activate; it also makes the contract more interesting… The diagram below gives a high level overview of the logic we want to implement.
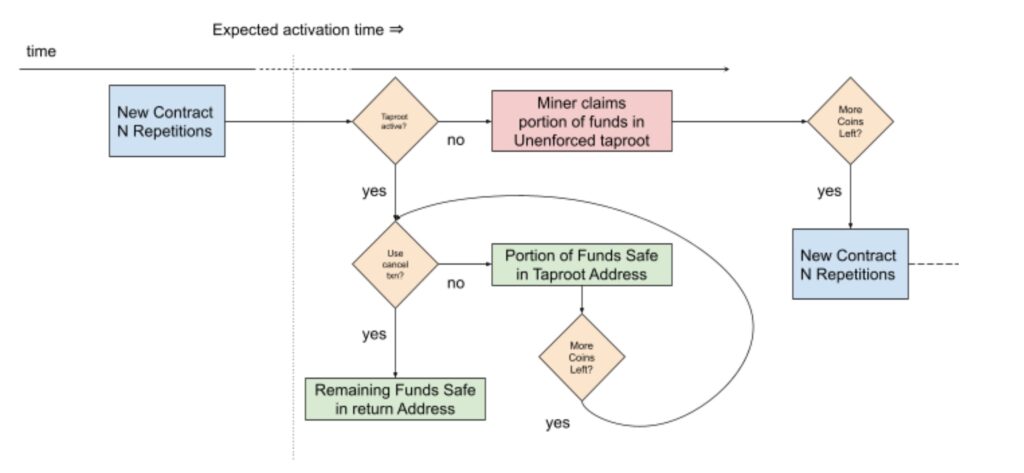
About 10 minutes after Madars texted me and I had a functional prototype that I refined the next morning.
Without further ado, let’s look at the code:
/// Taproot Recurring Bet.
/// This data structure captures all the arguments required to build a contract.
#[derive(JsonSchema, Serialize, Deserialize, Clone)]
pub struct TapBet {
/// How much Bitcoin to release per period
#[schemars(with = "f64")]
#[serde(with = "bitcoin::util::amount::serde::as_btc")]
pub amount_per_time: Amount,
/// How much in fees to pay per cycle.
/// TODO: In theory, this could be zero, as miners could manually add such
/// transactions (which they topet a reward out of) to their mempools.
/// TODO: Optional, make cancellation path have a different feerate
#[schemars(with = "f64")]
#[serde(with = "bitcoin::util::amount::serde::as_btc")]
pub fees_per_time: Amount,
/// How frequently should we test to see if Taproot is active?
pub period: AnyRelTimeLock,
/// How long to wait to allow early-abort of the contract unfolding (should
/// be > period)
pub cancel_timeout: AnyRelTimeLock,
/// An externally generated Taproot script (not address) to send the funds to
pub taproot_script: Script,
/// An arbitrary bitcoin address to send the funds to on cancellation
pub cancel_to: bitcoin::Address,
}
/// This defines the interface for the TapBet Contract
impl Contract for TapBet {
/// The "next steps" that can happen for an instance of a TapBet
/// is either to:
/// - stop_expansion: return the funds safely to the creator because Taproot is active
/// - continue_expansion: take amount_per_time of the funds and send them to a taproot address.
/// > If taproot is active, the funds are safe in that key
/// > If taproot is not active, a miner may steal the funds
declare! {then, Self::stop_expansion, Self::continue_expansion}
/// you can ignore this line, it is only needed for an advanced Sapio feature
/// and will be able to be removed when a specific rust feature stabilizes.
declare! {non updatable}
}
/// The actual logic for each TapBet
impl TapBet {
/// The waiting period is over, sample if Taproot is active
guard! {period_over |s, ctx| { s.period.into() }}
then! {continue_expansion [Self::period_over] |s, ctx| {
// creates a new transaction template for the next step
// of this contract
let mut builder = ctx.template().set_label("continue_expansion".into());
// set the sequence validly
builder = builder.set_sequence(0, s.period.into())?;
// if we have sufficient funds, pay out to a taproot address now
if builder.ctx().funds() >= s.amount_per_time {
let mut range = AmountRange::new();
range.update_range(s.amount_per_time);
builder = builder.add_output(
s.amount_per_time,
&Compiled::from_script(s.taproot_script.clone(), Some(range), ctx.network)?,
None
)?;
}
// if we have funds remaining, make a recursive TapBet with the same
// parameters.
if builder.ctx().funds() >= s.fees_per_time {
let amt =
builder.ctx().funds() - s.fees_per_time;
if amt > Amount::from_sat(0) {
builder = builder.add_output(
amt,
s,
None
)?;
}
}
builder.into()
}}
/// The timeout period is over
guard! {timeout |s, ctx| { s.cancel_timeout.into() }}
then! {stop_expansion [Self::timeout] |s, ctx| {
let mut builder = ctx.template().set_label("stop_expansion".into());
builder = builder.set_sequence(0, s.cancel_timeout.into())?;
// Pay out to the original owner
if builder.ctx().funds() >= s.fees_per_time {
let amt = builder.ctx().funds() - s.fees_per_time;
if amt > Amount::from_sat(0) {
builder = builder.add_output(
amt,
&Compiled::from_address(s.cancel_to.clone(), None),
None
)?;
}
}
builder.into()
}}
}
/// This registers our contract API with the module system.
REGISTER![TapBet];
The TapBet contract definition compiles into a WebAssembly module that can be loaded and used to make contract instances. For convenience, Sapio provides a Rust library / CLI for interfacing with compiled contract plugins. A contract can be created3 as follows:
cargo run --bin cli -- contract create 0.09015
'{"amount_per_time": 0.03,
"cancel_timeout": {"RH" : 2116},
"cancel_to": "bcrt1qs758ursh4q9z627kt3pp5yysm78ddny6txaqgw",
"fees_per_time": 0.00005,
"period": {"RH": 2016},
"taproot_script": "51200279BE667EF9DCBBAC55A06295CE870B07029BFCDB2DCE28D959F2815B16F817"}'
--file="plugin-example/pkg/sapio_wasm_plugin_example_bg.wasm"
Note the bitcoin amount passed in (0.09015) is sufficient for 3 iterations of 0.03 sized bets with 0.00005 in fees per bet. The contract steps mature every 2,016 blocks, and the contract may be cancelled 100 blocks after a miner has failed to expand the contract. This compiles to the output at the end of the article (see appendix 1). One simply pays 0.09015 Bitcoin to the address created (bcrt1qgc803gks4d89362ql09ayycr2s7k6v4ze34pc4ndc0ggu4j24lgqewwf7y) and then posts the created output with the contract JSON for others to verify the honest signal. These contract objects can be manipulated to be bound to a specific UTXO to create a PSBT list (see appendix 2) and rendered for display using the Tux interface (pictured above). The command’s output contains Sapio-specific metadata and annotations to assist in contract manipulations and processing, but at core every Sapio contract is a simple list of all possible transactions.
In the future, CheckTemplateVerify will guarantee that the contract executes faithfully. That is, the only ways the funds can move is via the pathways labelled “then!” and no other way. At this time, because CheckTemplateVerify is not yet available, we may emulate the functionality of CTV — albeit with a worsened security model — using a signing oracle server instead. In this specific contract, using a personal signing server would not reduce the behavior of the contract greatly because a rational miner would only select transactions which are more rewarding than proposed, so there is limited opportunity to cheat.
In order to cheat, a user would end up having to offer more money than is already available to the miners under the existing transaction. It’s possible that a user could break the honesty of the signal and bribe a miner with, say, 0.04 BTC to end the contract early and return the value used to signal in the remaining periods. There are a few ways to address this limitation:
- Only ever fund a single period of this contract (no recurring)
- Use a federation of third-party signatories (potentially in addition to your own key) so that you’d have to coerce the notary to let you cheat
- Activate OP_CTV, obviating the need for any signing servers.
With enough evidence in hand that a large economic aggregate of users plan to rely on Taproot activating by a certain time, the network would be foolish — and possibly economically forked — were it to not enforce the rule by that time.
So, should you rush out to go and make an honest signal that you want Taproot by a certain date? I’m not gonna stop you! But I’d advise caution — this is a new concept, and the game theory of giving a miner a reason to pretend they’ve never heard of “taproot” and steal your money may be problematic; it’s a total game of chicken. Further, activating on this basis would require broad ecosystem support to monitor and measure the amount placed in such contracts. The author’s opinion is the best bet at this juncture is to form consensus around one of the existing BIP8/9 family activations, but encourages readers at home to continue to tinker and think about the bleeding edge of smart contracts and consensus.
Want to try out Sapio and build your own smart contracts? Stay tuned! We’ll be releasing publicly soon.
Notes
1 – It is out of the scope of this article to go into depth on all the potential upgrade mechanisms, but for a summary of existing proposals, see Aaron van Wirdum’s article in Bitcoin Magazine.
2 – Winning coins is just a little trickier — it’s easier to spend money than to make money! Would someone really pay you to lose money to miners if Taproot doesn’t activate? One way to implement a premium is to make it so that the transaction creating the contract has a second output for a premium and the transaction is underfunded with SigHash AnyoneCanPay to add funds for the premium.
3 – The taproot_script parameter might seem magical, but it is just the script OP_1 PUSH32 <32 bytes pubkey> to create a V1 Segwit Output, see https://github.com/bitcoin/bips/blob/master/bip-0341.mediawiki for more details.
Appendices
Appendix 1: Contract JSON
{
"template_hash_to_template_map": {
"a6dbda05299a7225d6422e8a0d163b9e5c734143eb1a507a5772e65edb4898dd": {
"precomputed_template_hash": "a6dbda05299a7225d6422e8a0d163b9e5c734143eb1a507a5772e65edb4898dd",
"precomputed_template_hash_idx": 0,
"max_amount_sats": 9010000,
"metadata_map_s2s": {
"label": "stop_expansion"
},
"transaction_literal": {
"version": 2,
"lock_time": 0,
"input": [
{
"previous_output": "0000000000000000000000000000000000000000000000000000000000000000:4294967295",
"script_sig": "",
"sequence": 2116,
"witness": []
}
],
"output": [
{
"value": 9010000,
"script_pubkey": "001487a87e0e17a80a2d2bd65c421a1090df8ed6cc9a"
}
]
},
"outputs_info": [
{
"sending_amount_sats": 9010000,
"receiving_contract": {
"address": "bcrt1qs758ursh4q9z627kt3pp5yysm78ddny6txaqgw",
"amount_range": {
"max_btc": 21000000
}
}
}
]
},
"2a5a2eecaf8090ffc6668ae60f70722d8d4791f9c282c6da0ecc5e8838c38175": {
"precomputed_template_hash": "2a5a2eecaf8090ffc6668ae60f70722d8d4791f9c282c6da0ecc5e8838c38175",
"precomputed_template_hash_idx": 0,
"max_amount_sats": 9010000,
"metadata_map_s2s": {
"label": "continue_expansion"
},
"transaction_literal": {
"version": 2,
"lock_time": 0,
"input": [
{
"previous_output": "0000000000000000000000000000000000000000000000000000000000000000:4294967295",
"script_sig": "",
"sequence": 2016,
"witness": []
}
],
"output": [
{
"value": 3000000,
"script_pubkey": "51200279be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f817"
},
{
"value": 6010000,
"script_pubkey": "00206709d4f25ab69955961cc8538e5d58343ae3aa3709a3fd9c7c0fa33f4af63887"
}
]
},
"outputs_info": [
{
"sending_amount_sats": 3000000,
"receiving_contract": {
"address": "bcrt1pqfumuen7l8wthtz45p3ftn58pvrs9xlumvkuu2xet8egzkcklqtscyvky6",
"amount_range": {
"max_btc": 0.03
}
}
},
{
"sending_amount_sats": 6010000,
"receiving_contract": {
"template_hash_to_template_map": {
"d61ac7b03b54f0ad0ab9e9e69ad966e4faa325375577cb259e7891a7b493dc82": {
"precomputed_template_hash": "d61ac7b03b54f0ad0ab9e9e69ad966e4faa325375577cb259e7891a7b493dc82",
"precomputed_template_hash_idx": 0,
"max_amount_sats": 6005000,
"metadata_map_s2s": {
"label": "stop_expansion"
},
"transaction_literal": {
"version": 2,
"lock_time": 0,
"input": [
{
"previous_output": "0000000000000000000000000000000000000000000000000000000000000000:4294967295",
"script_sig": "",
"sequence": 2116,
"witness": []
}
],
"output": [
{
"value": 6005000,
"script_pubkey": "001487a87e0e17a80a2d2bd65c421a1090df8ed6cc9a"
}
]
},
"outputs_info": [
{
"sending_amount_sats": 6005000,
"receiving_contract": {
"address": "bcrt1qs758ursh4q9z627kt3pp5yysm78ddny6txaqgw",
"amount_range": {
"max_btc": 21000000
}
}
}
]
},
"ebcea40f3558db070bf30445c208b329e2386080662dd5d6fe29da8f0c301ffb": {
"precomputed_template_hash": "ebcea40f3558db070bf30445c208b329e2386080662dd5d6fe29da8f0c301ffb",
"precomputed_template_hash_idx": 0,
"max_amount_sats": 6005000,
"metadata_map_s2s": {
"label": "continue_expansion"
},
"transaction_literal": {
"version": 2,
"lock_time": 0,
"input": [
{
"previous_output": "0000000000000000000000000000000000000000000000000000000000000000:4294967295",
"script_sig": "",
"sequence": 2016,
"witness": []
}
],
"output": [
{
"value": 3000000,
"script_pubkey": "51200279be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f817"
},
{
"value": 3005000,
"script_pubkey": "0020d023b8ffc5162902a1902e12d0282957fb4726a0f83efc2cb7efc4a6d4247483"
}
]
},
"outputs_info": [
{
"sending_amount_sats": 3000000,
"receiving_contract": {
"address": "bcrt1pqfumuen7l8wthtz45p3ftn58pvrs9xlumvkuu2xet8egzkcklqtscyvky6",
"amount_range": {
"max_btc": 0.03
}
}
},
{
"sending_amount_sats": 3005000,
"receiving_contract": {
"template_hash_to_template_map": {
"64ca1a03d428ce2bb3413080cfa303dcdc841ba30900c2d72cd3615593bf294b": {
"precomputed_template_hash": "64ca1a03d428ce2bb3413080cfa303dcdc841ba30900c2d72cd3615593bf294b",
"precomputed_template_hash_idx": 0,
"max_amount_sats": 3000000,
"metadata_map_s2s": {
"label": "continue_expansion"
},
"transaction_literal": {
"version": 2,
"lock_time": 0,
"input": [
{
"previous_output": "0000000000000000000000000000000000000000000000000000000000000000:4294967295",
"script_sig": "",
"sequence": 2016,
"witness": []
}
],
"output": [
{
"value": 3000000,
"script_pubkey": "51200279be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f817"
}
]
},
"outputs_info": [
{
"sending_amount_sats": 3000000,
"receiving_contract": {
"address": "bcrt1pqfumuen7l8wthtz45p3ftn58pvrs9xlumvkuu2xet8egzkcklqtscyvky6",
"amount_range": {
"max_btc": 0.03
}
}
}
]
},
"037164a6bc5e23333ccd750972927c4de669face404841ce476b867eb08a5d1e": {
"precomputed_template_hash": "037164a6bc5e23333ccd750972927c4de669face404841ce476b867eb08a5d1e",
"precomputed_template_hash_idx": 0,
"max_amount_sats": 3000000,
"metadata_map_s2s": {
"label": "stop_expansion"
},
"transaction_literal": {
"version": 2,
"lock_time": 0,
"input": [
{
"previous_output": "0000000000000000000000000000000000000000000000000000000000000000:4294967295",
"script_sig": "",
"sequence": 2116,
"witness": []
}
],
"output": [
{
"value": 3000000,
"script_pubkey": "001487a87e0e17a80a2d2bd65c421a1090df8ed6cc9a"
}
]
},
"outputs_info": [
{
"sending_amount_sats": 3000000,
"receiving_contract": {
"address": "bcrt1qs758ursh4q9z627kt3pp5yysm78ddny6txaqgw",
"amount_range": {
"max_btc": 21000000
}
}
}
]
}
},
"known_policy": "thresh(1,and(older(2116),pk(02d9259ccb8d82d21b80155926dc8dd840f22a862792eba28c935b5221bda7f696)),and(older(2016),pk(037a7ffbdac37874b
4128c484318f3a9c0f0f2088628181d2ed83add9e4ab291c1)))",
"address": "bcrt1q6q3m3l79zc5s9gvs9cfdq2pf2la5wf4qlql0ct9halz2d4pywjpsa25fsl",
"known_descriptor": "wsh(thresh(1,nj:and_v(v:pk(02d9259ccb8d82d21b80155926dc8dd840f22a862792eba28c935b5221bda7f696),older(2116)),snj:and_v(v:pk(037a7f
fbdac37874b4128c484318f3a9c0f0f2088628181d2ed83add9e4ab291c1),older(2016))))#fcpsm54r",
"amount_range": {
"max_btc": 0.03
}
}
}
]
}
},
"known_policy": "thresh(1,and(older(2116),pk(03bac00d55195f4822f0d6660b43e2a0c20bb162542fe7656400024c499edb21a1)),and(older(2016),pk(03ea94374570b77c401224ffe33
5b4dd4a640e5cd7d52c3d2f9e5fe3a5b02e481e)))",
"address": "bcrt1qvuyafuj6k6v4t9suepfcuh2cxsaw823hpx3lm8rup73n7jhk8zrsmkaflr",
"known_descriptor": "wsh(thresh(1,nj:and_v(v:pk(03bac00d55195f4822f0d6660b43e2a0c20bb162542fe7656400024c499edb21a1),older(2116)),snj:and_v(v:pk(03ea94374570b77c
401224ffe335b4dd4a640e5cd7d52c3d2f9e5fe3a5b02e481e),older(2016))))#wc87daeg",
"amount_range": {
"max_btc": 0.06005
}
}
}
]
}
},
"known_policy": "thresh(1,and(older(2116),pk(02d3c32217f7bc3d43f1b8cfd7425379a3bf07074f1a3b4d243546e80e69b0c172)),and(older(2016),pk(02214f6ce3917541ca51705ea29e592dd4e39
cfffcf8f46d43e26011e331e6f5bd)))",
"address": "bcrt1qgc803gks4d89362ql09ayycr2s7k6v4ze34pc4ndc0ggu4j24lgqewwf7y",
"known_descriptor": "wsh(thresh(1,nj:and_v(v:pk(02d3c32217f7bc3d43f1b8cfd7425379a3bf07074f1a3b4d243546e80e69b0c172),older(2116)),snj:and_v(v:pk(02214f6ce3917541ca51705ea2
9e592dd4e39cfffcf8f46d43e26011e331e6f5bd),older(2016))))#nu55ucem",
"amount_range": {
"max_btc": 0.0901
}
}
Appendix 2: Contract PSBTs and MetaData
[
[
{
"global": {
"unsigned_tx": {
"version": 2,
"lock_time": 0,
"input": [
{
"previous_output": "4fac5f4526a712ad202029cb713469f15c65925be29ac52f89cb637d03b3b678:0",
"script_sig": "",
"sequence": 2016,
"witness": []
}
],
"output": [
{
"value": 3000000,
"script_pubkey": "51200279be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f817"
},
{
"value": 6010000,
"script_pubkey": "00206709d4f25ab69955961cc8538e5d58343ae3aa3709a3fd9c7c0fa33f4af63887"
}
]
},
"version": 0,
"xpub": {},
"proprietary": [],
"unknown": []
},
"inputs": [
{
"non_witness_utxo": null,
"witness_utxo": {
"value": 9010000,
"script_pubkey": "0020460ef8a2d0ab4e58e940fbcbd21303543d6d32a2cc6a1c566dc3d08e564aafd0"
},
"partial_sigs": {
"02214f6ce3917541ca51705ea29e592dd4e39cfffcf8f46d43e26011e331e6f5bd": "1ce7a3360f44340211df12a3fa98ef29b577cd2f4b228c0e2ff3
bc90f7659395711913e17c7ce033a60a3c7f957f64cc399f6d574767ca5206c3b8e6755ef7d001"
},
"sighash_type": "SIGHASH_ALL",
"redeem_script": null,
"witness_script": "8292632102d3c32217f7bc3d43f1b8cfd7425379a3bf07074f1a3b4d243546e80e69b0c172ad024408b268927c8292632102214f6c
e3917541ca51705ea29e592dd4e39cfffcf8f46d43e26011e331e6f5bdad02e007b26892935187",
"bip32_derivation": [],
"final_script_sig": null,
"final_script_witness": null,
"ripemd160_preimages": {},
"sha256_preimages": {},
"hash160_preimages": {},
"hash256_preimages": {},
"proprietary": [],
"unknown": []
}
],
"outputs": [
{
"redeem_script": null,
"witness_script": null,
"bip32_derivation": [],
"proprietary": [],
"unknown": []
},
{
"redeem_script": null,
"witness_script": null,
"bip32_derivation": [],
"proprietary": [],
"unknown": []
}
]
},
{
"global": {
"unsigned_tx": {
"version": 2,
"lock_time": 0,
"input": [
{
"previous_output": "4fac5f4526a712ad202029cb713469f15c65925be29ac52f89cb637d03b3b678:0",
"script_sig": "",
"sequence": 2116,
"witness": []
}
],
"output": [
{
"value": 9010000,
"script_pubkey": "001487a87e0e17a80a2d2bd65c421a1090df8ed6cc9a"
}
]
},
"version": 0,
"xpub": {},
"proprietary": [],
"unknown": []
},
"inputs": [
{
"non_witness_utxo": null,
"witness_utxo": {
"value": 9010000,
"script_pubkey": "0020460ef8a2d0ab4e58e940fbcbd21303543d6d32a2cc6a1c566dc3d08e564aafd0"
},
"partial_sigs": {
"02d3c32217f7bc3d43f1b8cfd7425379a3bf07074f1a3b4d243546e80e69b0c172": "e95b534aecc4325ade5c3241d06c4606de2244a990d01879b4d8
9370e31a3b9b2fe7f242505eb76915931de3a23b6f06d59a7f437c98cf083b84296859a5755b01"
},
"sighash_type": "SIGHASH_ALL",
"redeem_script": null,
"witness_script": "8292632102d3c32217f7bc3d43f1b8cfd7425379a3bf07074f1a3b4d243546e80e69b0c172ad024408b268927c8292632102214f6c
e3917541ca51705ea29e592dd4e39cfffcf8f46d43e26011e331e6f5bdad02e007b26892935187",
"bip32_derivation": [],
"final_script_sig": null,
"final_script_witness": null,
"ripemd160_preimages": {},
"sha256_preimages": {},
"hash160_preimages": {},
"hash256_preimages": {},
"proprietary": [],
"unknown": []
}
],
"outputs": [
{
"redeem_script": null,
"witness_script": null,
"bip32_derivation": [],
"proprietary": [],
"unknown": []
}
]
},
{
"global": {
"unsigned_tx": {
"version": 2,
"lock_time": 0,
"input": [
{
"previous_output": "d9b7e135a0265e77b7cab8c9254cbe2638bca168c00120dde56e41fb74e36d5a:1",
"script_sig": "",
"sequence": 2116,
"witness": []
}
],
"output": [
{
"value": 6005000,
"script_pubkey": "001487a87e0e17a80a2d2bd65c421a1090df8ed6cc9a"
}
]
},
"version": 0,
"xpub": {},
"proprietary": [],
"unknown": []
},
"inputs": [
{
"non_witness_utxo": null,
"witness_utxo": {
"value": 6010000,
"script_pubkey": "00206709d4f25ab69955961cc8538e5d58343ae3aa3709a3fd9c7c0fa33f4af63887"
},
"partial_sigs": {
"03bac00d55195f4822f0d6660b43e2a0c20bb162542fe7656400024c499edb21a1": "0f24413cf9e4a0d53ff24496969d357b5c0a95f25b61f98d9fda
de8a2c8c612a5dcc211242ff81fd3836c7adaa8a9c1988a5f48719d87b2fe1c34afb666c198601"
},
"sighash_type": "SIGHASH_ALL",
"redeem_script": null,
"witness_script": "8292632103bac00d55195f4822f0d6660b43e2a0c20bb162542fe7656400024c499edb21a1ad024408b268927c8292632103ea9437
4570b77c401224ffe335b4dd4a640e5cd7d52c3d2f9e5fe3a5b02e481ead02e007b26892935187",
"bip32_derivation": [],
"final_script_sig": null,
"final_script_witness": null,
"ripemd160_preimages": {},
"sha256_preimages": {},
"hash160_preimages": {},
"hash256_preimages": {},
"proprietary": [],
"unknown": []
}
],
"outputs": [
{
"redeem_script": null,
"witness_script": null,
"bip32_derivation": [],
"proprietary": [],
"unknown": []
}
]
},
{
"global": {
"unsigned_tx": {
"version": 2,
"lock_time": 0,
"input": [
{
"previous_output": "d9b7e135a0265e77b7cab8c9254cbe2638bca168c00120dde56e41fb74e36d5a:1",
"script_sig": "",
"sequence": 2016,
"witness": []
}
],
"output": [
{
"value": 3000000,
"script_pubkey": "51200279be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f817"
},
{
"value": 3005000,
"script_pubkey": "0020d023b8ffc5162902a1902e12d0282957fb4726a0f83efc2cb7efc4a6d4247483"
}
]
},
"version": 0,
"xpub": {},
"proprietary": [],
"unknown": []
},
"inputs": [
{
"non_witness_utxo": null,
"witness_utxo": {
"value": 6010000,
"script_pubkey": "00206709d4f25ab69955961cc8538e5d58343ae3aa3709a3fd9c7c0fa33f4af63887"
},
"partial_sigs": {
"03ea94374570b77c401224ffe335b4dd4a640e5cd7d52c3d2f9e5fe3a5b02e481e": "7fef952d3488420612e2ff5bb422606112357085b7dcb745534e
59c31db9795076f54f9173448a0ffd7f93f98139ce0f155583427356b77f5da8be69393a67f301"
},
"sighash_type": "SIGHASH_ALL",
"redeem_script": null,
"witness_script": "8292632103bac00d55195f4822f0d6660b43e2a0c20bb162542fe7656400024c499edb21a1ad024408b268927c8292632103ea9437
4570b77c401224ffe335b4dd4a640e5cd7d52c3d2f9e5fe3a5b02e481ead02e007b26892935187",
"bip32_derivation": [],
"final_script_sig": null,
"final_script_witness": null,
"ripemd160_preimages": {},
"sha256_preimages": {},
"hash160_preimages": {},
"hash256_preimages": {},
"proprietary": [],
"unknown": []
}
],
"outputs": [
{
"redeem_script": null,
"witness_script": null,
"bip32_derivation": [],
"proprietary": [],
"unknown": []
},
{
"redeem_script": null,
"witness_script": null,
"bip32_derivation": [],
"proprietary": [],
"unknown": []
}
]
},
{
"global": {
"unsigned_tx": {
"version": 2,
"lock_time": 0,
"input": [
{
"previous_output": "e3b104521048350140ffb52914cc780fe45a44c733e2524a25ec1a79c235639b:1",
"script_sig": "",
"sequence": 2116,
"witness": []
}
],
"output": [
{
"value": 3000000,
"script_pubkey": "001487a87e0e17a80a2d2bd65c421a1090df8ed6cc9a"
}
]
},
"version": 0,
"xpub": {},
"proprietary": [],
"unknown": []
},
"inputs": [
{
"non_witness_utxo": null,
"witness_utxo": {
"value": 3005000,
"script_pubkey": "0020d023b8ffc5162902a1902e12d0282957fb4726a0f83efc2cb7efc4a6d4247483"
},
"partial_sigs": {
"02d9259ccb8d82d21b80155926dc8dd840f22a862792eba28c935b5221bda7f696": "58848a5e8abb37ac1c395ec5934e984bd25496488a0ca718ce28
2ce742686a247156f7c42a84b07db71f1d265ef9ee3dcab34491ee62cac6d6d064cb3abf87a201"
},
"sighash_type": "SIGHASH_ALL",
"redeem_script": null,
"witness_script": "8292632102d9259ccb8d82d21b80155926dc8dd840f22a862792eba28c935b5221bda7f696ad024408b268927c82926321037a7ffb
dac37874b4128c484318f3a9c0f0f2088628181d2ed83add9e4ab291c1ad02e007b26892935187",
"bip32_derivation": [],
"final_script_sig": null,
"final_script_witness": null,
"ripemd160_preimages": {},
"sha256_preimages": {},
"hash160_preimages": {},
"hash256_preimages": {},
"proprietary": [],
"unknown": []
}
],
"outputs": [
{
"redeem_script": null,
"witness_script": null,
"bip32_derivation": [],
"proprietary": [],
"unknown": []
}
]
},
{
"global": {
"unsigned_tx": {
"version": 2,
"lock_time": 0,
"input": [
{
"previous_output": "e3b104521048350140ffb52914cc780fe45a44c733e2524a25ec1a79c235639b:1",
"script_sig": "",
"sequence": 2016,
"witness": []
}
],
"output": [
{
"value": 3000000,
"script_pubkey": "51200279be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f817"
}
]
},
"version": 0,
"xpub": {},
"proprietary": [],
"unknown": []
},
"inputs": [
{
"non_witness_utxo": null,
"witness_utxo": {
"value": 3005000,
"script_pubkey": "0020d023b8ffc5162902a1902e12d0282957fb4726a0f83efc2cb7efc4a6d4247483"
},
"partial_sigs": {
"037a7ffbdac37874b4128c484318f3a9c0f0f2088628181d2ed83add9e4ab291c1": "ad0cb6da9994d8b1ae6aea2e158c728887d5f3c9a57bfbc0346d
85ffdf30769e370fb72b1067fc7cb62e7350a5624b2a07e376baa8ec0e769b0187d08663ec9c01"
},
"sighash_type": "SIGHASH_ALL",
"redeem_script": null,
"witness_script": "8292632102d9259ccb8d82d21b80155926dc8dd840f22a862792eba28c935b5221bda7f696ad024408b268927c82926321037a7ffb
dac37874b4128c484318f3a9c0f0f2088628181d2ed83add9e4ab291c1ad02e007b26892935187",
"bip32_derivation": [],
"final_script_sig": null,
"final_script_witness": null,
"ripemd160_preimages": {},
"sha256_preimages": {},
"hash160_preimages": {},
"hash256_preimages": {},
"proprietary": [],
"unknown": []
}
],
"outputs": [
{
"redeem_script": null,
"witness_script": null,
"bip32_derivation": [],
"proprietary": [],
"unknown": []
}
]
},
{
"global": {
"unsigned_tx": {
"version": 2,
"lock_time": 0,
"input": [
{
"previous_output": "0a9038bbb488729f23c0c1d7c6cfa98ea8464f204fcd9f8d5d90ab91a995d2ba:0",
"script_sig": "",
"sequence": 4294967294,
"witness": []
}
],
"output": [
{
"value": 9010000,
"script_pubkey": "0020460ef8a2d0ab4e58e940fbcbd21303543d6d32a2cc6a1c566dc3d08e564aafd0"
},
{
"value": 190986940,
"script_pubkey": "0014b423d53997e1607cf64e31a5a107360fa69db3bd"
}
]
},
"version": 0,
"xpub": {},
"proprietary": [],
"unknown": []
},
"inputs": [
{
"non_witness_utxo": null,
"witness_utxo": null,
"partial_sigs": {},
"sighash_type": null,
"redeem_script": null,
"witness_script": null,
"bip32_derivation": [],
"final_script_sig": null,
"final_script_witness": null,
"ripemd160_preimages": {},
"sha256_preimages": {},
"hash160_preimages": {},
"hash256_preimages": {},
"proprietary": [],
"unknown": []
}
],
"outputs": [
{
"redeem_script": null,
"witness_script": null,
"bip32_derivation": [],
"proprietary": [],
"unknown": []
},
{
"redeem_script": null,
"witness_script": null,
"bip32_derivation": [],
"proprietary": [],
"unknown": []
}
]
}
],
[
{
"color": "green",
"metadata": {
"label": "continue_expansion"
},
"utxo_metadata": [
{},
{}
]
},
{
"color": "green",
"metadata": {
"label": "stop_expansion"
},
"utxo_metadata": [
{}
]
},
{
"color": "green",
"metadata": {
"label": "stop_expansion"
},
"utxo_metadata": [
{}
]
},
{
"color": "green",
"metadata": {
"label": "continue_expansion"
},
"utxo_metadata": [
{},
{}
]
},
{
"color": "green",
"metadata": {
"label": "stop_expansion"
},
"utxo_metadata": [
{}
]
},
{
"color": "green",
"metadata": {
"label": "continue_expansion"
},
"utxo_metadata": [
{}
]
},
{
"color": "black",
"metadata": {
"label": "funding"
},
"utxo_metadata": {}
}
]
]